Euclid Q1: cloud access#
Learning Goals#
By the end of this tutorial, you will:
Learn where Euclid Q1 data are stored in the cloud.
Retrieve an image cutout from the cloud.
Retrieve a spectrum from the cloud.
1. Introduction#
Euclid launched in July 2023 as a European Space Agency (ESA) mission with involvement by NASA. The primary science goals of Euclid are to better understand the composition and evolution of the dark Universe. The Euclid mission is providing space-based imaging and spectroscopy as well as supporting ground-based imaging to achieve these primary goals. These data will be archived by multiple global repositories, including IRSA, where they will support transformational work in many areas of astrophysics.
Euclid Quick Release 1 (Q1) consists of consists of ~30 TB of imaging, spectroscopy, and catalogs covering four non-contiguous fields: Euclid Deep Field North (22.9 sq deg), Euclid Deep Field Fornax (12.1 sq deg), Euclid Deep Field South (28.1 sq deg), and LDN1641.
IRSA maintains copies of the Euclid Q1 data products both on premises at IPAC and on the cloud via Amazon Web Services (AWS). This notebook provides an introduction to accessing Euclid Q1 data from the cloud. If you have questions, please contact the IRSA helpdesk.
2. Imports#
s3fs
for browsing S3 bucketsastropy
for handling coordinates, units, FITS I/O, tables, images, etc.astroquery>=0.4.10
for querying Euclid data products from IRSAmatplotlib
for visualizationjson
for decoding JSON strings
Important
We rely on astroquery
features that have been recently added, so please make sure you have version v0.4.10 or newer installed.
# Uncomment the next line to install dependencies if needed.
# !pip install s3fs astropy 'astroquery>=0.4.10' matplotlib
import s3fs
from astropy.coordinates import SkyCoord
import astropy.units as u
from astropy.visualization import ImageNormalize, PercentileInterval, AsinhStretch
from astropy.io import fits
from astropy.nddata import Cutout2D
from astropy.wcs import WCS
from astropy.table import Table
from astroquery.ipac.irsa import Irsa
from matplotlib import pyplot as plt
import json
3. Browse Euclid Q1 cloud-hosted data#
BUCKET_NAME = 'nasa-irsa-euclid-q1'
s3fs provides a filesystem-like python interface for AWS S3 buckets. First we create a s3 client:
s3 = s3fs.S3FileSystem(anon=True)
Then we list the q1
directory that contains Euclid Q1 data products:
s3.ls(f'{BUCKET_NAME}/q1')
['nasa-irsa-euclid-q1/q1/MER',
'nasa-irsa-euclid-q1/q1/MER_SEG',
'nasa-irsa-euclid-q1/q1/NIR',
'nasa-irsa-euclid-q1/q1/RAW',
'nasa-irsa-euclid-q1/q1/SIR',
'nasa-irsa-euclid-q1/q1/VIS',
'nasa-irsa-euclid-q1/q1/VMPZ',
'nasa-irsa-euclid-q1/q1/catalogs']
Let’s navigate to MER images (available as FITS files):
s3.ls(f'{BUCKET_NAME}/q1/MER')[:10] # ls only top 10 to limit the long output
['nasa-irsa-euclid-q1/q1/MER/102018211',
'nasa-irsa-euclid-q1/q1/MER/102018212',
'nasa-irsa-euclid-q1/q1/MER/102018213',
'nasa-irsa-euclid-q1/q1/MER/102018664',
'nasa-irsa-euclid-q1/q1/MER/102018665',
'nasa-irsa-euclid-q1/q1/MER/102018666',
'nasa-irsa-euclid-q1/q1/MER/102018667',
'nasa-irsa-euclid-q1/q1/MER/102018668',
'nasa-irsa-euclid-q1/q1/MER/102018669',
'nasa-irsa-euclid-q1/q1/MER/102018670']
s3.ls(f'{BUCKET_NAME}/q1/MER/102018211') # pick any tile ID from above
['nasa-irsa-euclid-q1/q1/MER/102018211/DECAM',
'nasa-irsa-euclid-q1/q1/MER/102018211/NISP',
'nasa-irsa-euclid-q1/q1/MER/102018211/VIS']
s3.ls(f'{BUCKET_NAME}/q1/MER/102018211/VIS') # pick any instrument from above
['nasa-irsa-euclid-q1/q1/MER/102018211/VIS/EUC_MER_BGMOD-VIS_TILE102018211-4CD9D_20241018T142710.276652Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/MER/102018211/VIS/EUC_MER_BGSUB-MOSAIC-VIS_TILE102018211-ACBD03_20241018T142710.276838Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/MER/102018211/VIS/EUC_MER_CATALOG-PSF-VIS_TILE102018211-55C665_20241018T204235.689380Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/MER/102018211/VIS/EUC_MER_GRID-PSF-VIS_TILE102018211-9A5C08_20241018T142525.109781Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/MER/102018211/VIS/EUC_MER_MOSAIC-VIS-FLAG_TILE102018211-40A124_20241018T142525.109767Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/MER/102018211/VIS/EUC_MER_MOSAIC-VIS-RMS_TILE102018211-B3070B_20241018T142525.109753Z_00.00.fits']
As per “Browsable Directories” section in user guide, we need MER/{tile_id}/{instrument}/EUC_MER_BGSUB-MOSAIC*.fits
for displaying background-subtracted mosiac images. But these images are stored under TILE IDs so first we need to find TILE ID for a coordinate search we are interested in. We will use astroquery (in next section) to retrieve FITS file paths for our coordinates by doing spatial search.
4. Do a spatial search for MER mosaics#
Pick a target and search radius:
target_name = 'TYC 4429-1677-1'
coord = SkyCoord.from_name(target_name)
search_radius = 10 * u.arcsec
As per “Data Products Overview” in user guide and above table, we identify that MER Mosiacs are available as the following collection:
img_collection = 'euclid_DpdMerBksMosaic'
Now query this collection for our target’s coordinates and search radius:
img_tbl = Irsa.query_sia(pos=(coord, search_radius), collection=img_collection)
img_tbl
s_ra | s_dec | facility_name | instrument_name | dataproduct_subtype | calib_level | dataproduct_type | energy_bandpassname | energy_emband | obs_id | s_resolution | em_min | em_max | em_res_power | proposal_title | access_url | access_format | access_estsize | t_exptime | s_region | obs_collection | obs_intent | algorithm_name | facility_keywords | instrument_keywords | environment_photometric | proposal_id | proposal_pi | proposal_project | target_name | target_type | target_standard | target_moving | target_keywords | obs_release_date | s_xel1 | s_xel2 | s_pixel_scale | position_timedependent | t_min | t_max | t_resolution | t_xel | obs_publisher_did | s_fov | em_xel | pol_states | pol_xel | cloud_access | o_ucd | upload_row_id |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
deg | deg | arcsec | m | m | kbyte | s | deg | arcsec | d | d | s | deg | ||||||||||||||||||||||||||||||||||||||
float64 | float64 | object | object | object | int16 | object | object | object | object | float64 | float64 | float64 | float64 | object | object | object | int64 | float64 | object | object | object | object | object | object | bool | object | object | object | object | object | bool | bool | object | object | int64 | int64 | float64 | bool | float64 | float64 | float64 | int64 | object | float64 | int64 | object | int64 | object | object | int64 |
273.07162262404495 | 68.00001388888474 | Euclid | NISP | noise | 3 | image | Y | Infrared | 102160339_NISP | 0.0878 | 9.2e-07 | 1.146e-06 | 4.6 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/NISP/EUC_MER_MOSAIC-NIR-Y-RMS_TILE102160339-975287_20241024T223523.501123Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_NISP/Y | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/NISP/EUC_MER_MOSAIC-NIR-Y-RMS_TILE102160339-975287_20241024T223523.501123Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Euclid | NISP | science | 3 | image | Y | Infrared | 102160339_NISP | 0.0878 | 9.2e-07 | 1.146e-06 | 4.6 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-Y_TILE102160339-3A9BC7_20241024T231706.521789Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_NISP/Y | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-Y_TILE102160339-3A9BC7_20241024T231706.521789Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Euclid | NISP | auxiliary | 3 | image | Y | Infrared | 102160339_NISP | 0.0878 | 9.2e-07 | 1.146e-06 | 4.6 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/NISP/EUC_MER_CATALOG-PSF-NIR-Y_TILE102160339-E72766_20241025T072629.031850Z_00.00.fits | image/fits | 549787 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_NISP/Y | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/NISP/EUC_MER_CATALOG-PSF-NIR-Y_TILE102160339-E72766_20241025T072629.031850Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Euclid | NISP | auxiliary | 3 | image | Y | Infrared | 102160339_NISP | 0.0878 | 9.2e-07 | 1.146e-06 | 4.6 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/NISP/EUC_MER_GRID-PSF-NIR-Y_TILE102160339-3306DF_20241024T223523.501156Z_00.00.fits | image/fits | 1475188 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_NISP/Y | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/NISP/EUC_MER_GRID-PSF-NIR-Y_TILE102160339-3306DF_20241024T223523.501156Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Euclid | NISP | auxiliary | 3 | image | Y | Infrared | 102160339_NISP | 0.0878 | 9.2e-07 | 1.146e-06 | 4.6 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/NISP/EUC_MER_BGMOD-NIR-Y_TILE102160339-83950E_20241024T231706.521466Z_00.00.fits | image/fits | 1474563 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_NISP/Y | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/NISP/EUC_MER_BGMOD-NIR-Y_TILE102160339-83950E_20241024T231706.521466Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Euclid | NISP | auxiliary | 3 | image | Y | Infrared | 102160339_NISP | 0.0878 | 9.2e-07 | 1.146e-06 | 4.6 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/NISP/EUC_MER_MOSAIC-NIR-Y-FLAG_TILE102160339-257E27_20241024T223523.501139Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_NISP/Y | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/NISP/EUC_MER_MOSAIC-NIR-Y-FLAG_TILE102160339-257E27_20241024T223523.501139Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | CFHT | MegaCam | science | 3 | image | U | Optical | 102160339_MegaCam | -- | 3.14751e-07 | 4.01839e-07 | 4.1 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/MEGACAM/EUC_MER_BGSUB-MOSAIC-CFIS-U_TILE102160339-B76075_20241024T224001.853984Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_MegaCam/U | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/MEGACAM/EUC_MER_BGSUB-MOSAIC-CFIS-U_TILE102160339-B76075_20241024T224001.853984Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | CFHT | MegaCam | auxiliary | 3 | image | U | Optical | 102160339_MegaCam | -- | 3.14751e-07 | 4.01839e-07 | 4.1 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/MEGACAM/EUC_MER_BGMOD-CFIS-U_TILE102160339-B46410_20241024T224001.853720Z_00.00.fits | image/fits | 1474563 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_MegaCam/U | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/MEGACAM/EUC_MER_BGMOD-CFIS-U_TILE102160339-B46410_20241024T224001.853720Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | CFHT | MegaCam | auxiliary | 3 | image | U | Optical | 102160339_MegaCam | -- | 3.14751e-07 | 4.01839e-07 | 4.1 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/MEGACAM/EUC_MER_MOSAIC-CFIS-U-FLAG_TILE102160339-FB39D_20241024T222309.855577Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_MegaCam/U | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/MEGACAM/EUC_MER_MOSAIC-CFIS-U-FLAG_TILE102160339-FB39D_20241024T222309.855577Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
273.07162262404495 | 68.00001388888474 | Subaru Telescope | Hyper Suprime-Cam | science | 3 | image | Z | Optical | 102160339_WISHES | 1.15 | 8.36385e-07 | 9.49057e-07 | 7.9 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/HSC/EUC_MER_BGSUB-MOSAIC-WISHES-Z_TILE102160339-50455D_20241024T223629.659159Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_WISHES/Z | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/HSC/EUC_MER_BGSUB-MOSAIC-WISHES-Z_TILE102160339-50455D_20241024T223629.659159Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Subaru Telescope | Hyper Suprime-Cam | noise | 3 | image | Z | Optical | 102160339_WISHES | 1.15 | 8.36385e-07 | 9.49057e-07 | 7.9 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/HSC/EUC_MER_MOSAIC-WISHES-Z-RMS_TILE102160339-D3B0D9_20241024T222306.537259Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_WISHES/Z | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/HSC/EUC_MER_MOSAIC-WISHES-Z-RMS_TILE102160339-D3B0D9_20241024T222306.537259Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Subaru Telescope | Hyper Suprime-Cam | auxiliary | 3 | image | Z | Optical | 102160339_WISHES | 1.15 | 8.36385e-07 | 9.49057e-07 | 7.9 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/HSC/EUC_MER_BGMOD-WISHES-Z_TILE102160339-F48933_20241024T223629.659029Z_00.00.fits | image/fits | 1474563 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_WISHES/Z | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/HSC/EUC_MER_BGMOD-WISHES-Z_TILE102160339-F48933_20241024T223629.659029Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Subaru Telescope | Hyper Suprime-Cam | auxiliary | 3 | image | Z | Optical | 102160339_WISHES | 1.15 | 8.36385e-07 | 9.49057e-07 | 7.9 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/HSC/EUC_MER_MOSAIC-WISHES-Z-FLAG_TILE102160339-383905_20241024T222306.537289Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_WISHES/Z | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/HSC/EUC_MER_MOSAIC-WISHES-Z-FLAG_TILE102160339-383905_20241024T222306.537289Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Subaru Telescope | Hyper Suprime-Cam | auxiliary | 3 | image | G | Optical | 102160339_WISHES | 1.58 | 3.95422e-07 | 5.54729e-07 | 3.0 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/HSC/EUC_MER_CATALOG-PSF-WISHES-G_TILE102160339-3FBE37_20241024T222302.191374Z_00.00.fits | image/fits | 549150 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_WISHES/G | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/HSC/EUC_MER_CATALOG-PSF-WISHES-G_TILE102160339-3FBE37_20241024T222302.191374Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Subaru Telescope | Hyper Suprime-Cam | auxiliary | 3 | image | G | Optical | 102160339_WISHES | 1.58 | 3.95422e-07 | 5.54729e-07 | 3.0 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/HSC/EUC_MER_BGMOD-WISHES-G_TILE102160339-809205_20241024T224427.004630Z_00.00.fits | image/fits | 1474563 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_WISHES/G | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/HSC/EUC_MER_BGMOD-WISHES-G_TILE102160339-809205_20241024T224427.004630Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Subaru Telescope | Hyper Suprime-Cam | auxiliary | 3 | image | G | Optical | 102160339_WISHES | 1.58 | 3.95422e-07 | 5.54729e-07 | 3.0 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/HSC/EUC_MER_MOSAIC-WISHES-G-FLAG_TILE102160339-7F63CF_20241024T222302.191361Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_WISHES/G | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/HSC/EUC_MER_MOSAIC-WISHES-G-FLAG_TILE102160339-7F63CF_20241024T222302.191361Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Subaru Telescope | Hyper Suprime-Cam | science | 3 | image | G | Optical | 102160339_WISHES | 1.58 | 3.95422e-07 | 5.54729e-07 | 3.0 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/HSC/EUC_MER_BGSUB-MOSAIC-WISHES-G_TILE102160339-3B55EE_20241024T224427.004846Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_WISHES/G | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/HSC/EUC_MER_BGSUB-MOSAIC-WISHES-G_TILE102160339-3B55EE_20241024T224427.004846Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Subaru Telescope | Hyper Suprime-Cam | noise | 3 | image | G | Optical | 102160339_WISHES | 1.58 | 3.95422e-07 | 5.54729e-07 | 3.0 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/HSC/EUC_MER_MOSAIC-WISHES-G-RMS_TILE102160339-5EB01E_20241024T222302.191348Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_WISHES/G | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/HSC/EUC_MER_MOSAIC-WISHES-G-RMS_TILE102160339-5EB01E_20241024T222302.191348Z_00.00.fits", "region": "us-east-1"}} | 1 |
Let’s narrow it down to the images with science dataproduct subtype and Euclid facility:
euclid_sci_img_tbl = img_tbl[[row['facility_name']=='Euclid'
and row['dataproduct_subtype']=='science'
for row in img_tbl]]
euclid_sci_img_tbl
s_ra | s_dec | facility_name | instrument_name | dataproduct_subtype | calib_level | dataproduct_type | energy_bandpassname | energy_emband | obs_id | s_resolution | em_min | em_max | em_res_power | proposal_title | access_url | access_format | access_estsize | t_exptime | s_region | obs_collection | obs_intent | algorithm_name | facility_keywords | instrument_keywords | environment_photometric | proposal_id | proposal_pi | proposal_project | target_name | target_type | target_standard | target_moving | target_keywords | obs_release_date | s_xel1 | s_xel2 | s_pixel_scale | position_timedependent | t_min | t_max | t_resolution | t_xel | obs_publisher_did | s_fov | em_xel | pol_states | pol_xel | cloud_access | o_ucd | upload_row_id |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
deg | deg | arcsec | m | m | kbyte | s | deg | arcsec | d | d | s | deg | ||||||||||||||||||||||||||||||||||||||
float64 | float64 | object | object | object | int16 | object | object | object | object | float64 | float64 | float64 | float64 | object | object | object | int64 | float64 | object | object | object | object | object | object | bool | object | object | object | object | object | bool | bool | object | object | int64 | int64 | float64 | bool | float64 | float64 | float64 | int64 | object | float64 | int64 | object | int64 | object | object | int64 |
273.07162262404495 | 68.00001388888474 | Euclid | NISP | science | 3 | image | Y | Infrared | 102160339_NISP | 0.0878 | 9.2e-07 | 1.146e-06 | 4.6 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-Y_TILE102160339-3A9BC7_20241024T231706.521789Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_NISP/Y | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-Y_TILE102160339-3A9BC7_20241024T231706.521789Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Euclid | VIS | science | 3 | image | VIS | Optical | 102160339_VIS | 0.16 | 5.5e-07 | 9e-07 | 2.1 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/VIS/EUC_MER_BGSUB-MOSAIC-VIS_TILE102160339-ADF1FF_20241025T022657.824470Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_VIS/VIS | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/VIS/EUC_MER_BGSUB-MOSAIC-VIS_TILE102160339-ADF1FF_20241025T022657.824470Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Euclid | NISP | science | 3 | image | J | Infrared | 102160339_NISP | 0.094 | 1.146e-06 | 1.372e-06 | 5.6 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-J_TILE102160339-B6EC5E_20241024T230609.320185Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_NISP/J | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-J_TILE102160339-B6EC5E_20241024T230609.320185Z_00.00.fits", "region": "us-east-1"}} | 1 | |||||||||
273.07162262404495 | 68.00001388888474 | Euclid | NISP | science | 3 | image | H | Infrared | 102160339_NISP | 0.1026 | 1.372e-06 | 2e-06 | 2.7 | Euclid on-the-fly | https://irsa.ipac.caltech.edu/ibe/data/euclid/q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-H_TILE102160339-539320_20241024T230405.104628Z_00.00.fits | image/fits | 1474566 | -- | POLYGON ICRS 273.7917384920759 68.26512213776137 272.3515059037093 68.2651218134747 272.3679070260387 67.73183349848344 273.7753390775804 67.73183381419538 273.7917384920759 68.26512213776137 | euclid_DpdMerBksMosaic | SCIENCE | mosaic | -- | field | -- | False | 2025-05-01 00:00:00 | 19200 | 19200 | 0.100000000000008 | False | -- | -- | -- | -- | ivo://irsa.ipac/euclid_DpdMerBksMosaic?102160339_NISP/H | 0.533333333333376 | -- | -- | {"aws": {"bucket_name": "nasa-irsa-euclid-q1", "key":"q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-H_TILE102160339-539320_20241024T230405.104628Z_00.00.fits", "region": "us-east-1"}} | 1 |
We can see there’s a cloud_access
column that gives us the location info of the image files we are interested in. So let’s extract the S3 bucket file path from it:
def get_s3_fpath(cloud_access):
cloud_info = json.loads(cloud_access) # converts str to dict
bucket_name = cloud_info['aws']['bucket_name']
key = cloud_info['aws']['key']
return f'{bucket_name}/{key}'
[get_s3_fpath(row['cloud_access']) for row in euclid_sci_img_tbl]
['nasa-irsa-euclid-q1/q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-Y_TILE102160339-3A9BC7_20241024T231706.521789Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/MER/102160339/VIS/EUC_MER_BGSUB-MOSAIC-VIS_TILE102160339-ADF1FF_20241025T022657.824470Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-J_TILE102160339-B6EC5E_20241024T230609.320185Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/MER/102160339/NISP/EUC_MER_BGSUB-MOSAIC-NIR-H_TILE102160339-539320_20241024T230405.104628Z_00.00.fits']
Let’s also extract filter names to use when displaying the images:
def get_filter_name(instrument, bandpass):
return f'{instrument}_{bandpass}' if instrument!=bandpass else instrument
[get_filter_name(row['instrument_name'], row['energy_bandpassname']) for row in euclid_sci_img_tbl]
['NISP_Y', 'VIS', 'NISP_J', 'NISP_H']
5. Efficiently retrieve mosaic cutouts#
These image files are very big (~1.4GB), so we use astropy’s lazy-loading capability of FITS for better performance. (See Obtaining subsets from cloud-hosted FITS files.)
cutout_size = 1 * u.arcmin
cutouts = []
filters = []
for row in euclid_sci_img_tbl:
s3_fpath = get_s3_fpath(row['cloud_access'])
filter_name = get_filter_name(row['instrument_name'], row['energy_bandpassname'])
with fits.open(f's3://{s3_fpath}', fsspec_kwargs={"anon": True}) as hdul:
print(f'Retrieving cutout for {filter_name} ...')
cutout = Cutout2D(hdul[0].section,
position=coord,
size=cutout_size,
wcs=WCS(hdul[0].header))
cutouts.append(cutout)
filters.append(filter_name)
Retrieving cutout for NISP_Y ...
Retrieving cutout for VIS ...
Retrieving cutout for NISP_J ...
Retrieving cutout for NISP_H ...
fig, axes = plt.subplots(2, 2, figsize=(4 * 2, 4 * 2), subplot_kw={'projection': cutouts[0].wcs})
for idx, ax in enumerate(axes.flat):
norm = ImageNormalize(cutouts[idx].data, interval=PercentileInterval(99), stretch=AsinhStretch())
ax.imshow(cutouts[idx].data, cmap='gray', origin='lower', norm=norm)
ax.set_xlabel('RA')
ax.set_ylabel('Dec')
ax.text(0.95, 0.05, filters[idx], color='white', fontsize=14, transform=ax.transAxes, va='bottom', ha='right')
plt.tight_layout()
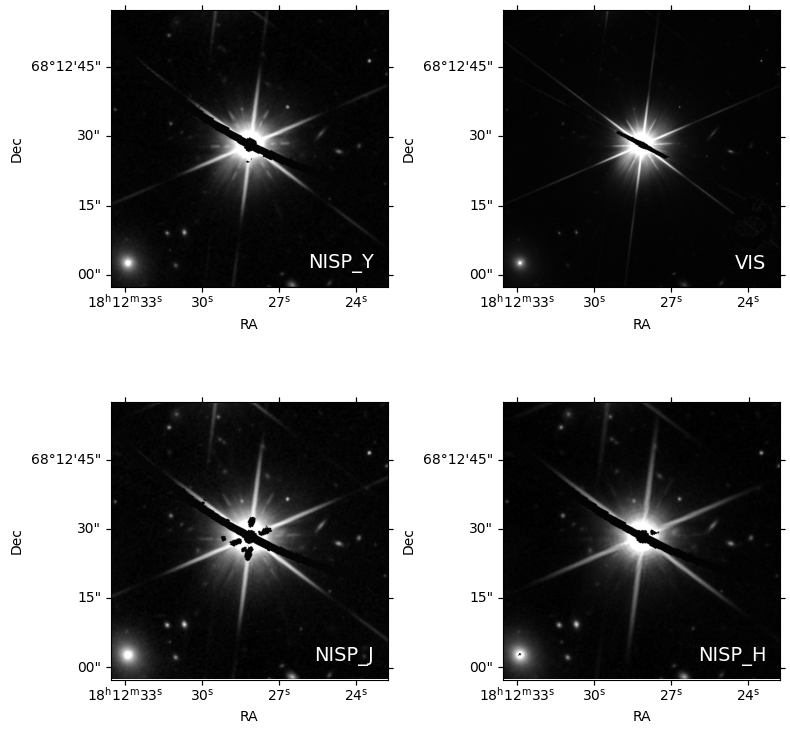
6. Find the MER catalog for a given tile#
Let’s navigate to MER catalog in the Euclid Q1 bucket:
s3.ls(f'{BUCKET_NAME}/q1/catalogs')
['nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG',
'nasa-irsa-euclid-q1/q1/catalogs/NIR_CAL_CATALOG',
'nasa-irsa-euclid-q1/q1/catalogs/PHZ_PF_OUTPUT_CATALOG',
'nasa-irsa-euclid-q1/q1/catalogs/PHZ_PF_OUTPUT_FOR_L3',
'nasa-irsa-euclid-q1/q1/catalogs/SPE_PF_OUTPUT_CATALOG',
'nasa-irsa-euclid-q1/q1/catalogs/VIS_CAL_CATALOG']
s3.ls(f'{BUCKET_NAME}/q1/catalogs/MER_FINAL_CATALOG')[:10] # ls only top 10 to limit the long output
['nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018211',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018212',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018213',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018664',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018665',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018666',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018667',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018668',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018669',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102018670']
mer_tile_id = 102160339 # from the image paths for the target we picked
s3.ls(f'{BUCKET_NAME}/q1/catalogs/MER_FINAL_CATALOG/{mer_tile_id}')
['nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102160339/EUC_MER_FINAL-CAT_TILE102160339-52AA9D_20241026T145149.163369Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102160339/EUC_MER_FINAL-CUTOUTS-CAT_TILE102160339-7449E7_20241026T034412.641243Z_00.00.fits',
'nasa-irsa-euclid-q1/q1/catalogs/MER_FINAL_CATALOG/102160339/EUC_MER_FINAL-MORPH-CAT_TILE102160339-52C86_20241026T145146.071857Z_00.00.fits']
As per “Browsable Directiories” section in user guide, we can use catalogs/MER_FINAL_CATALOG/{tile_id}/EUC_MER_FINAL-CAT*.fits
for listing the objects catalogued. We can read the identified FITS file as table and do filtering on ra, dec columns to find object ID(s) only for the target we picked. But it will be an expensive operation so we will instead use astroquery (in next section) to do a spatial search in the MER catalog provided by IRSA.
Note
Once the catalogs are available as Parquet files in the cloud, we can efficiently do spatial filtering directly on the cloud-hosted file to identify object ID(s) for our target. But for the time being, we can use catalog VO services through astroquery to do the same.
7. Find the MER Object ID for our target#
First, list the Euclid catalogs provided by IRSA:
catalogs = Irsa.list_catalogs(full=True, filter='euclid')
catalogs
table_index | schema_name | table_name | description | table_type | utype | irsa_dbms | irsa_pos | irsa_sptlevel | irsa_missionid | irsa_groupid | irsa_access_flag | irsa_nrows | irsa_odbc_datasource | irsa_spatial_idx_name |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
int32 | object | object | object | object | object | int32 | object | int32 | int32 | int32 | int32 | int64 | object | object |
938 | euclid | euclid_q1_mer_catalogue | Euclid Q1 MER Catalog | table | 21 | y | 20 | -1 | -1 | 30 | 29953430 | euclid | NIX_EUCLID_Q1_MER_CATALOGUE_HTM20 | |
939 | euclid | euclid_q1_mer_morphology | Euclid Q1 MER Morphology | table | 21 | n | 20 | -1 | -1 | 30 | 29953430 | euclid | ||
940 | euclid | euclid_q1_mer_cutouts | Euclid Q1 MER Cutouts | table | 21 | n | 20 | -1 | -1 | 30 | 29953430 | euclid | ||
941 | euclid | euclid_q1_phz_photo_z | Euclid Q1 PHZ Photo-z Catalog | table | 21 | n | 20 | -1 | -1 | 30 | 29643313 | euclid | ||
942 | euclid | euclid_q1_phz_star_sed | Euclid Q1 PHZ Star SED Catalog | table | 21 | n | 20 | -1 | -1 | 30 | 29187029 | euclid | ||
943 | euclid | euclid_q1_phz_galaxy_sed | Euclid Q1 PHZ Galaxy SED Catalog | table | 21 | n | 20 | -1 | -1 | 30 | 29437743 | euclid | ||
944 | euclid | euclid_q1_phz_classification | Euclid Q1 PHZ Classification Catalog | table | 21 | n | 20 | -1 | -1 | 30 | 29643313 | euclid | ||
945 | euclid | euclid_q1_phz_qso_physical_parameters | Euclid Q1 PHZ QSO Physical Parameters Catalog | table | 21 | n | 20 | -1 | -1 | 30 | 1442648 | euclid | ||
946 | euclid | euclid_q1_phz_nir_physical_parameters | Euclid Q1 PHZ NIR Physical Parameters Catalog | table | 21 | n | 20 | -1 | -1 | 30 | 7248114 | euclid | ||
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
954 | euclid | euclid_q1_spe_lines_continuum_features | Euclid Q1 SPE Lines Catalog - Continuum Features | table | 21 | n | 20 | -1 | -1 | 30 | 6133772 | euclid | ||
955 | euclid | euclid_q1_spe_lines_atomic_indices | Euclid Q1 SPE Lines Catalog - Atomic Indices | table | 21 | n | 20 | -1 | -1 | 30 | 110407896 | euclid | ||
956 | euclid | euclid_q1_spe_lines_molecular_indices | Euclid Q1 SPE Lines Catalog - Molecular Indices | table | 21 | n | 20 | -1 | -1 | 30 | 18401316 | euclid | ||
957 | euclid | euclid_q1_spectro_model_catalog_spe_lines_catalog | Euclid Q1 SPE Models Catalog - Lines | table | 21 | n | 20 | -1 | -1 | 30 | 37376 | euclid | ||
958 | euclid | euclid_q1_spectro_model_catalog_spe_star_models | Euclid Q1 SPE Star Models Catalog | table | 21 | n | 20 | -1 | -1 | 30 | 6545058 | euclid | ||
959 | euclid | euclid.tileid_association_q1 | Euclid Q1 TILEID to Observation ID Association Table | table | 22 | n | 20 | -1 | -1 | 30 | 7572 | euclid_postgres | ||
960 | euclid | euclid.objectid_spectrafile_association_q1 | Euclid Q1 Object ID to Spectral File Association Table | table | 22 | n | 20 | -1 | -1 | 30 | 29952509 | euclid_postgres | ||
961 | euclid | euclid.observation_euclid_q1 | Euclid Q1 CAOM Observation Table | view | 22 | n | 20 | -1 | -1 | 30 | 1420 | euclid_postgres | ||
962 | euclid | euclid.plane_euclid_q1 | Euclid Q1 CAOM Plane Table | view | 22 | y | 20 | -1 | -1 | 30 | 4525 | euclid_postgres | ||
963 | euclid | euclid.artifact_euclid_q1 | Euclid Q1 CAOM Artifact Table | view | 22 | n | 20 | -1 | -1 | 30 | 37519 | euclid_postgres |
From this table, we can extract the MER catalog name. We also see several other interesting catalogs, let’s also extract spectral file association catalog for retrieving spectra later.
euclid_mer_catalog = 'euclid_q1_mer_catalogue'
euclid_spec_association_catalog = 'euclid.objectid_spectrafile_association_q1'
Now, we do a region search within a cone of 5 arcsec around our target to pinpoint its object ID in Euclid catalog:
search_radius = 5 * u.arcsec
mer_catalog_tbl = Irsa.query_region(coordinates=coord, spatial='Cone',
catalog=euclid_mer_catalog, radius=search_radius)
mer_catalog_tbl
object_id | ra | dec | right_ascension_psf_fitting | declination_psf_fitting | segmentation_map_id | vis_det | flux_vis_1fwhm_aper | flux_vis_2fwhm_aper | flux_vis_3fwhm_aper | flux_vis_4fwhm_aper | flux_y_1fwhm_aper | flux_y_2fwhm_aper | flux_y_3fwhm_aper | flux_y_4fwhm_aper | flux_j_1fwhm_aper | flux_j_2fwhm_aper | flux_j_3fwhm_aper | flux_j_4fwhm_aper | flux_h_1fwhm_aper | flux_h_2fwhm_aper | flux_h_3fwhm_aper | flux_h_4fwhm_aper | flux_nir_stack_1fwhm_aper | flux_nir_stack_2fwhm_aper | flux_nir_stack_3fwhm_aper | flux_nir_stack_4fwhm_aper | flux_u_ext_decam_1fwhm_aper | flux_u_ext_decam_2fwhm_aper | flux_u_ext_decam_3fwhm_aper | flux_u_ext_decam_4fwhm_aper | flux_g_ext_decam_1fwhm_aper | flux_g_ext_decam_2fwhm_aper | flux_g_ext_decam_3fwhm_aper | flux_g_ext_decam_4fwhm_aper | flux_r_ext_decam_1fwhm_aper | flux_r_ext_decam_2fwhm_aper | flux_r_ext_decam_3fwhm_aper | flux_r_ext_decam_4fwhm_aper | flux_i_ext_decam_1fwhm_aper | flux_i_ext_decam_2fwhm_aper | flux_i_ext_decam_3fwhm_aper | flux_i_ext_decam_4fwhm_aper | flux_z_ext_decam_1fwhm_aper | flux_z_ext_decam_2fwhm_aper | flux_z_ext_decam_3fwhm_aper | flux_z_ext_decam_4fwhm_aper | flux_u_ext_lsst_1fwhm_aper | flux_u_ext_lsst_2fwhm_aper | flux_u_ext_lsst_3fwhm_aper | flux_u_ext_lsst_4fwhm_aper | flux_g_ext_lsst_1fwhm_aper | flux_g_ext_lsst_2fwhm_aper | flux_g_ext_lsst_3fwhm_aper | flux_g_ext_lsst_4fwhm_aper | flux_r_ext_lsst_1fwhm_aper | flux_r_ext_lsst_2fwhm_aper | flux_r_ext_lsst_3fwhm_aper | flux_r_ext_lsst_4fwhm_aper | flux_i_ext_lsst_1fwhm_aper | flux_i_ext_lsst_2fwhm_aper | flux_i_ext_lsst_3fwhm_aper | flux_i_ext_lsst_4fwhm_aper | flux_z_ext_lsst_1fwhm_aper | flux_z_ext_lsst_2fwhm_aper | flux_z_ext_lsst_3fwhm_aper | flux_z_ext_lsst_4fwhm_aper | flux_u_ext_megacam_1fwhm_aper | flux_u_ext_megacam_2fwhm_aper | flux_u_ext_megacam_3fwhm_aper | flux_u_ext_megacam_4fwhm_aper | flux_r_ext_megacam_1fwhm_aper | flux_r_ext_megacam_2fwhm_aper | flux_r_ext_megacam_3fwhm_aper | flux_r_ext_megacam_4fwhm_aper | flux_g_ext_jpcam_1fwhm_aper | flux_g_ext_jpcam_2fwhm_aper | flux_g_ext_jpcam_3fwhm_aper | flux_g_ext_jpcam_4fwhm_aper | flux_i_ext_panstarrs_1fwhm_aper | flux_i_ext_panstarrs_2fwhm_aper | flux_i_ext_panstarrs_3fwhm_aper | flux_i_ext_panstarrs_4fwhm_aper | flux_z_ext_panstarrs_1fwhm_aper | flux_z_ext_panstarrs_2fwhm_aper | flux_z_ext_panstarrs_3fwhm_aper | flux_z_ext_panstarrs_4fwhm_aper | flux_g_ext_hsc_1fwhm_aper | flux_g_ext_hsc_2fwhm_aper | flux_g_ext_hsc_3fwhm_aper | flux_g_ext_hsc_4fwhm_aper | flux_z_ext_hsc_1fwhm_aper | flux_z_ext_hsc_2fwhm_aper | flux_z_ext_hsc_3fwhm_aper | flux_z_ext_hsc_4fwhm_aper | fluxerr_vis_1fwhm_aper | fluxerr_vis_2fwhm_aper | fluxerr_vis_3fwhm_aper | fluxerr_vis_4fwhm_aper | fluxerr_y_1fwhm_aper | fluxerr_y_2fwhm_aper | fluxerr_y_3fwhm_aper | fluxerr_y_4fwhm_aper | fluxerr_j_1fwhm_aper | fluxerr_j_2fwhm_aper | fluxerr_j_3fwhm_aper | fluxerr_j_4fwhm_aper | fluxerr_h_1fwhm_aper | fluxerr_h_2fwhm_aper | fluxerr_h_3fwhm_aper | fluxerr_h_4fwhm_aper | fluxerr_nir_stack_1fwhm_aper | fluxerr_nir_stack_2fwhm_aper | fluxerr_nir_stack_3fwhm_aper | fluxerr_nir_stack_4fwhm_aper | fluxerr_u_ext_decam_1fwhm_aper | fluxerr_u_ext_decam_2fwhm_aper | fluxerr_u_ext_decam_3fwhm_aper | fluxerr_u_ext_decam_4fwhm_aper | fluxerr_g_ext_decam_1fwhm_aper | fluxerr_g_ext_decam_2fwhm_aper | fluxerr_g_ext_decam_3fwhm_aper | fluxerr_g_ext_decam_4fwhm_aper | fluxerr_r_ext_decam_1fwhm_aper | fluxerr_r_ext_decam_2fwhm_aper | fluxerr_r_ext_decam_3fwhm_aper | fluxerr_r_ext_decam_4fwhm_aper | fluxerr_i_ext_decam_1fwhm_aper | fluxerr_i_ext_decam_2fwhm_aper | fluxerr_i_ext_decam_3fwhm_aper | fluxerr_i_ext_decam_4fwhm_aper | fluxerr_z_ext_decam_1fwhm_aper | fluxerr_z_ext_decam_2fwhm_aper | fluxerr_z_ext_decam_3fwhm_aper | fluxerr_z_ext_decam_4fwhm_aper | fluxerr_u_ext_lsst_1fwhm_aper | fluxerr_u_ext_lsst_2fwhm_aper | fluxerr_u_ext_lsst_3fwhm_aper | fluxerr_u_ext_lsst_4fwhm_aper | fluxerr_g_ext_lsst_1fwhm_aper | fluxerr_g_ext_lsst_2fwhm_aper | fluxerr_g_ext_lsst_3fwhm_aper | fluxerr_g_ext_lsst_4fwhm_aper | fluxerr_r_ext_lsst_1fwhm_aper | fluxerr_r_ext_lsst_2fwhm_aper | fluxerr_r_ext_lsst_3fwhm_aper | fluxerr_r_ext_lsst_4fwhm_aper | fluxerr_i_ext_lsst_1fwhm_aper | fluxerr_i_ext_lsst_2fwhm_aper | fluxerr_i_ext_lsst_3fwhm_aper | fluxerr_i_ext_lsst_4fwhm_aper | fluxerr_z_ext_lsst_1fwhm_aper | fluxerr_z_ext_lsst_2fwhm_aper | fluxerr_z_ext_lsst_3fwhm_aper | fluxerr_z_ext_lsst_4fwhm_aper | fluxerr_u_ext_megacam_1fwhm_aper | fluxerr_u_ext_megacam_2fwhm_aper | fluxerr_u_ext_megacam_3fwhm_aper | fluxerr_u_ext_megacam_4fwhm_aper | fluxerr_r_ext_megacam_1fwhm_aper | fluxerr_r_ext_megacam_2fwhm_aper | fluxerr_r_ext_megacam_3fwhm_aper | fluxerr_r_ext_megacam_4fwhm_aper | fluxerr_g_ext_jpcam_1fwhm_aper | fluxerr_g_ext_jpcam_2fwhm_aper | fluxerr_g_ext_jpcam_3fwhm_aper | fluxerr_g_ext_jpcam_4fwhm_aper | fluxerr_i_ext_panstarrs_1fwhm_aper | fluxerr_i_ext_panstarrs_2fwhm_aper | fluxerr_i_ext_panstarrs_3fwhm_aper | fluxerr_i_ext_panstarrs_4fwhm_aper | fluxerr_z_ext_panstarrs_1fwhm_aper | fluxerr_z_ext_panstarrs_2fwhm_aper | fluxerr_z_ext_panstarrs_3fwhm_aper | fluxerr_z_ext_panstarrs_4fwhm_aper | fluxerr_g_ext_hsc_1fwhm_aper | fluxerr_g_ext_hsc_2fwhm_aper | fluxerr_g_ext_hsc_3fwhm_aper | fluxerr_g_ext_hsc_4fwhm_aper | fluxerr_z_ext_hsc_1fwhm_aper | fluxerr_z_ext_hsc_2fwhm_aper | fluxerr_z_ext_hsc_3fwhm_aper | fluxerr_z_ext_hsc_4fwhm_aper | flux_y_templfit | flux_j_templfit | flux_h_templfit | flux_u_ext_decam_templfit | flux_g_ext_decam_templfit | flux_r_ext_decam_templfit | flux_i_ext_decam_templfit | flux_z_ext_decam_templfit | flux_u_ext_lsst_templfit | flux_g_ext_lsst_templfit | flux_r_ext_lsst_templfit | flux_i_ext_lsst_templfit | flux_z_ext_lsst_templfit | flux_u_ext_megacam_templfit | flux_r_ext_megacam_templfit | flux_g_ext_jpcam_templfit | flux_i_ext_panstarrs_templfit | flux_z_ext_panstarrs_templfit | flux_g_ext_hsc_templfit | flux_z_ext_hsc_templfit | fluxerr_y_templfit | fluxerr_j_templfit | fluxerr_h_templfit | fluxerr_u_ext_decam_templfit | fluxerr_g_ext_decam_templfit | fluxerr_r_ext_decam_templfit | fluxerr_i_ext_decam_templfit | fluxerr_z_ext_decam_templfit | fluxerr_u_ext_lsst_templfit | fluxerr_g_ext_lsst_templfit | fluxerr_r_ext_lsst_templfit | fluxerr_i_ext_lsst_templfit | fluxerr_z_ext_lsst_templfit | fluxerr_u_ext_megacam_templfit | fluxerr_r_ext_megacam_templfit | fluxerr_g_ext_jpcam_templfit | fluxerr_i_ext_panstarrs_templfit | fluxerr_z_ext_panstarrs_templfit | fluxerr_g_ext_hsc_templfit | fluxerr_z_ext_hsc_templfit | flux_vis_to_y_templfit | flux_vis_to_j_templfit | flux_vis_to_h_templfit | flux_vis_to_u_ext_decam_templfit | flux_vis_to_g_ext_decam_templfit | flux_vis_to_r_ext_decam_templfit | flux_vis_to_i_ext_decam_templfit | flux_vis_to_z_ext_decam_templfit | flux_vis_to_u_ext_lsst_templfit | flux_vis_to_g_ext_lsst_templfit | flux_vis_to_r_ext_lsst_templfit | flux_vis_to_i_ext_lsst_templfit | flux_vis_to_z_ext_lsst_templfit | flux_vis_to_u_ext_megacam_templfit | flux_vis_to_r_ext_megacam_templfit | flux_vis_to_g_ext_jpcam_templfit | flux_vis_to_i_ext_panstarrs_templfit | flux_vis_to_z_ext_panstarrs_templfit | flux_vis_to_g_ext_hsc_templfit | flux_vis_to_z_ext_hsc_templfit | fluxerr_vis_to_y_templfit | fluxerr_vis_to_j_templfit | fluxerr_vis_to_h_templfit | fluxerr_vis_to_u_ext_decam_templfit | fluxerr_vis_to_g_ext_decam_templfit | fluxerr_vis_to_r_ext_decam_templfit | fluxerr_vis_to_i_ext_decam_templfit | fluxerr_vis_to_z_ext_decam_templfit | fluxerr_vis_to_u_ext_lsst_templfit | fluxerr_vis_to_g_ext_lsst_templfit | fluxerr_vis_to_r_ext_lsst_templfit | fluxerr_vis_to_i_ext_lsst_templfit | fluxerr_vis_to_z_ext_lsst_templfit | fluxerr_vis_to_u_ext_megacam_templfit | fluxerr_vis_to_r_ext_megacam_templfit | fluxerr_vis_to_g_ext_jpcam_templfit | fluxerr_vis_to_i_ext_panstarrs_templfit | fluxerr_vis_to_z_ext_panstarrs_templfit | fluxerr_vis_to_g_ext_hsc_templfit | fluxerr_vis_to_z_ext_hsc_templfit | flux_vis_psf | fluxerr_vis_psf | flux_segmentation | fluxerr_segmentation | flux_detection_total | fluxerr_detection_total | flux_vis_sersic | flux_y_sersic | flux_j_sersic | flux_h_sersic | flux_u_ext_decam_sersic | flux_g_ext_decam_sersic | flux_r_ext_decam_sersic | flux_i_ext_decam_sersic | flux_z_ext_decam_sersic | flux_u_ext_lsst_sersic | flux_g_ext_lsst_sersic | flux_r_ext_lsst_sersic | flux_i_ext_lsst_sersic | flux_z_ext_lsst_sersic | flux_u_ext_megacam_sersic | flux_r_ext_megacam_sersic | flux_g_ext_jpcam_sersic | flux_i_ext_panstarrs_sersic | flux_z_ext_panstarrs_sersic | flux_g_ext_hsc_sersic | flux_z_ext_hsc_sersic | fluxerr_vis_sersic | fluxerr_y_sersic | fluxerr_j_sersic | fluxerr_h_sersic | fluxerr_u_ext_decam_sersic | fluxerr_g_ext_decam_sersic | fluxerr_r_ext_decam_sersic | fluxerr_i_ext_decam_sersic | fluxerr_z_ext_decam_sersic | fluxerr_u_ext_lsst_sersic | fluxerr_g_ext_lsst_sersic | fluxerr_r_ext_lsst_sersic | fluxerr_i_ext_lsst_sersic | fluxerr_z_ext_lsst_sersic | fluxerr_u_ext_megacam_sersic | fluxerr_r_ext_megacam_sersic | fluxerr_g_ext_jpcam_sersic | fluxerr_i_ext_panstarrs_sersic | fluxerr_z_ext_panstarrs_sersic | fluxerr_g_ext_hsc_sersic | fluxerr_z_ext_hsc_sersic | flux_vis_disk_sersic | flux_y_disk_sersic | flux_j_disk_sersic | flux_h_disk_sersic | flux_u_ext_decam_disk_sersic | flux_g_ext_decam_disk_sersic | flux_r_ext_decam_disk_sersic | flux_i_ext_decam_disk_sersic | flux_z_ext_decam_disk_sersic | flux_u_ext_lsst_disk_sersic | flux_g_ext_lsst_disk_sersic | flux_r_ext_lsst_disk_sersic | flux_i_ext_lsst_disk_sersic | flux_z_ext_lsst_disk_sersic | flux_u_ext_megacam_disk_sersic | flux_r_ext_megacam_disk_sersic | flux_g_ext_jpcam_disk_sersic | flux_i_ext_panstarrs_disk_sersic | flux_z_ext_panstarrs_disk_sersic | flux_g_ext_hsc_disk_sersic | flux_z_ext_hsc_disk_sersic | fluxerr_vis_disk_sersic | fluxerr_y_disk_sersic | fluxerr_j_disk_sersic | fluxerr_h_disk_sersic | fluxerr_u_ext_decam_disk_sersic | fluxerr_g_ext_decam_disk_sersic | fluxerr_r_ext_decam_disk_sersic | fluxerr_i_ext_decam_disk_sersic | fluxerr_z_ext_decam_disk_sersic | fluxerr_u_ext_lsst_disk_sersic | fluxerr_g_ext_lsst_disk_sersic | fluxerr_r_ext_lsst_disk_sersic | fluxerr_i_ext_lsst_disk_sersic | fluxerr_z_ext_lsst_disk_sersic | fluxerr_u_ext_megacam_disk_sersic | fluxerr_r_ext_megacam_disk_sersic | fluxerr_g_ext_jpcam_disk_sersic | fluxerr_i_ext_panstarrs_disk_sersic | fluxerr_z_ext_panstarrs_disk_sersic | fluxerr_g_ext_hsc_disk_sersic | fluxerr_z_ext_hsc_disk_sersic | sersic_fract_vis_disk_sersic | sersic_fract_y_disk_sersic | sersic_fract_j_disk_sersic | sersic_fract_h_disk_sersic | sersic_fract_u_ext_decam_disk_sersic | sersic_fract_g_ext_decam_disk_sersic | sersic_fract_r_ext_decam_disk_sersic | sersic_fract_i_ext_decam_disk_sersic | sersic_fract_z_ext_decam_disk_sersic | sersic_fract_u_ext_lsst_disk_sersic | sersic_fract_g_ext_lsst_disk_sersic | sersic_fract_r_ext_lsst_disk_sersic | sersic_fract_i_ext_lsst_disk_sersic | sersic_fract_z_ext_lsst_disk_sersic | sersic_fract_u_ext_megacam_disk_sersic | sersic_fract_r_ext_megacam_disk_sersic | sersic_fract_g_ext_jpcam_disk_sersic | sersic_fract_i_ext_panstarrs_disk_sersic | sersic_fract_z_ext_panstarrs_disk_sersic | sersic_fract_g_ext_hsc_disk_sersic | sersic_fract_z_ext_hsc_disk_sersic | sersic_fract_vis_disk_sersic_err | sersic_fract_y_disk_sersic_err | sersic_fract_j_disk_sersic_err | sersic_fract_h_disk_sersic_err | sersic_fract_u_ext_decam_disk_sersic_err | sersic_fract_g_ext_decam_disk_sersic_err | sersic_fract_r_ext_decam_disk_sersic_err | sersic_fract_i_ext_decam_disk_sersic_err | sersic_fract_z_ext_decam_disk_sersic_err | sersic_fract_u_ext_lsst_disk_sersic_err | sersic_fract_g_ext_lsst_disk_sersic_err | sersic_fract_r_ext_lsst_disk_sersic_err | sersic_fract_i_ext_lsst_disk_sersic_err | sersic_fract_z_ext_lsst_disk_sersic_err | sersic_fract_u_ext_megacam_disk_sersic_err | sersic_fract_r_ext_megacam_disk_sersic_err | sersic_fract_g_ext_jpcam_disk_sersic_err | sersic_fract_i_ext_panstarrs_disk_sersic_err | sersic_fract_z_ext_panstarrs_disk_sersic_err | sersic_fract_g_ext_hsc_disk_sersic_err | sersic_fract_z_ext_hsc_disk_sersic_err | flag_vis | flag_y | flag_j | flag_h | flag_nir_stack | flag_u_ext_decam | flag_g_ext_decam | flag_r_ext_decam | flag_i_ext_decam | flag_z_ext_decam | flag_u_ext_lsst | flag_g_ext_lsst | flag_r_ext_lsst | flag_i_ext_lsst | flag_z_ext_lsst | flag_u_ext_megacam | flag_r_ext_megacam | flag_g_ext_jpcam | flag_i_ext_panstarrs | flag_z_ext_panstarrs | flag_g_ext_hsc | flag_z_ext_hsc | avg_trans_wave_vis | avg_trans_wave_y | avg_trans_wave_j | avg_trans_wave_h | avg_trans_wave_u_ext_decam | avg_trans_wave_g_ext_decam | avg_trans_wave_r_ext_decam | avg_trans_wave_i_ext_decam | avg_trans_wave_z_ext_decam | avg_trans_wave_u_ext_lsst | avg_trans_wave_g_ext_lsst | avg_trans_wave_r_ext_lsst | avg_trans_wave_i_ext_lsst | avg_trans_wave_z_ext_lsst | avg_trans_wave_u_ext_megacam | avg_trans_wave_r_ext_megacam | avg_trans_wave_g_ext_jpcam | avg_trans_wave_i_ext_panstarrs | avg_trans_wave_z_ext_panstarrs | avg_trans_wave_g_ext_hsc | avg_trans_wave_z_ext_hsc | deblended_flag | parent_id | parent_visnir | blended_prob | she_flag | variable_flag | binary_flag | point_like_flag | point_like_prob | extended_flag | extended_prob | spurious_flag | spurious_prob | mag_stargal_sep | det_quality_flag | mu_max | mumax_minus_mag | segmentation_area | semimajor_axis | semimajor_axis_err | position_angle | position_angle_err | ellipticity | ellipticity_err | kron_radius | kron_radius_err | fwhm | gal_ebv | gal_ebv_err | gaia_id | gaia_match_quality | tileid | x | y | z | spt_ind | htm20 | cntr |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
deg | deg | deg | deg | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | uJy | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | Angstrom | mag | mag/arcsec**2 | mag/arcsec**2 | pix | pix | pix | deg | deg | pix | pix | arcsec | mag | mag | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
int64 | float64 | float64 | float64 | float64 | int64 | int64 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | int64 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | int64 | int64 | int64 | float32 | int64 | int64 | int64 | int64 | float32 | int64 | float32 | int64 | float32 | float32 | int64 | float32 | float32 | int64 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | float32 | int64 | float32 | int64 | float64 | float64 | float64 | int64 | int64 | int64 |
2731173428682078045 | 273.11734290 | 68.20780450 | -- | -- | 102160339092326 | 1 | -- | -- | -- | 15.80938244 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 4875.361328 | 12928.96191 | 16977.68164 | 18710.50977 | 1233.40625 | 4855.16748 | 10355.6709 | 15934.21387 | -- | -- | -- | -- | -- | -- | -- | 11.59000015 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 1495.251343 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 0.06763066351 | 0.1376733333 | 0.208141461 | 0.2812566459 | 0.03858586401 | 0.07920518517 | 0.1201112196 | 0.1619603336 | -- | -- | -- | -- | -- | -- | -- | 1.58172226 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 12848.43457 | 14002.1875 | 13081.42188 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 40729.17969 | 26445.17188 | -- | 30498.35547 | -- | 5874.598145 | 11243.15332 | 7.811558723 | 8.388196945 | 6.875566006 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 0.4524695873 | 0.2596883774 | -- | 8.57169342 | -- | 0.46092996 | 2.583146334 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 833.5445557 | 1.333409071 | 9262.958984 | 2.157295942 | 9372.352539 | 2.387297392 | 9373.876953 | 9372.282227 | 9372.175781 | 9371.804688 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 3327.687988 | 16259.23633 | -- | 46777.24219 | -- | 8571.042969 | 16432.23633 | 78.16717529 | 69.32390594 | 67.96690369 | 56.01578903 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 28.17465782 | 29.72841835 | -- | 230.1270752 | -- | 22.21575165 | 76.60475159 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 2 | 2 | 2 | 2 | 2 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 2 | 2 | -- | 2 | -- | 2 | 2 | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | -- | 3682.77002 | 6425.697754 | -- | 7535.640625 | -- | 4730.214844 | 8908.526367 | 1 | 6823 | 2722 | -- | -- | -- | -- | -- | -- | -- | -- | 0 | 0.02359147929 | 13.97037888 | 390 | 14.81733608 | 0.8469572067 | 110334 | 46.18929672 | -- | -54.62298584 | -- | 0.1192200407 | -- | 323.3251038 | -- | 1.295664072 | 0.06533759832 | 0.0008336739265 | 2258237767800082176 | 0.00388439768 | 102160339 | 0.0201884979526048 | -0.3706920167072900 | 0.9285364038635749 | 201320002 | 13709724288024 | 29269339 |
object_id = int(mer_catalog_tbl['object_id'][0])
object_id
2731173428682078045
8. Find the spectrum of an object in the MER catalog#
Using the object ID(s) we extracted above, we can narrow down the spectral file association catalog to identify spectra file path(s). So we do the following TAP search:
adql_query = f"SELECT * FROM {euclid_spec_association_catalog} \
WHERE objectid = {object_id}"
spec_association_tbl = Irsa.query_tap(adql_query).to_table()
spec_association_tbl
objectid | tileid | uri | hdu | cntr |
---|---|---|---|---|
int64 | int64 | object | int64 | int64 |
2731173428682078045 | 102160339 | ibe/data/euclid/q1/SIR/102160339/EUC_SIR_W-COMBSPEC_102160339_2024-11-05T16:26:34.614296Z.fits | 1644 | 3602837 |
Warning
If you picked a target other than what this notebook uses, it’s possible that there is no spectrum associated for your target’s object ID. In that case, spec_association_tbl
will contain 0 rows.
In above table, we can see that the uri
column gives us location of spectra file on IBE. We can map it to S3 bucket key to retrieve spectra file from the cloud. This is a very big FITS spectra file with multiple extensions where each extension contains spectrum of one object. The hdu
column gives us the extension number for our object. So let’s extract both of these.
spec_fpath_key = spec_association_tbl['uri'][0].replace('ibe/data/euclid/', '')
spec_fpath_key
'q1/SIR/102160339/EUC_SIR_W-COMBSPEC_102160339_2024-11-05T16:26:34.614296Z.fits'
object_hdu_idx = int(spec_association_tbl['hdu'][0])
object_hdu_idx
1644
Again, we use astropy’s lazy-loading capability of FITS to only retrieve the spectrum table of our object from the S3 bucket.
with fits.open(f's3://{BUCKET_NAME}/{spec_fpath_key}', fsspec_kwargs={'anon': True}) as hdul:
spec_hdu = hdul[object_hdu_idx]
spec_tbl = Table.read(spec_hdu)
WARNING: UnitsWarning: 'Number' did not parse as fits unit: At col 0, Unit 'Number' not supported by the FITS standard. If this is meant to be a custom unit, define it with 'u.def_unit'. To have it recognized inside a file reader or other code, enable it with 'u.add_enabled_units'. For details, see https://docs.astropy.org/en/latest/units/combining_and_defining.html [astropy.units.core]
WARNING: UnitsWarning: 'Number' did not parse as fits unit: At col 0, Unit 'Number' not supported by the FITS standard. If this is meant to be a custom unit, define it with 'u.def_unit'. To have it recognized inside a file reader or other code, enable it with 'u.add_enabled_units'. For details, see https://docs.astropy.org/en/latest/units/combining_and_defining.html [astropy.units.core]
WARNING: UnitsWarning: 'Number' did not parse as fits unit: At col 0, Unit 'Number' not supported by the FITS standard. If this is meant to be a custom unit, define it with 'u.def_unit'. To have it recognized inside a file reader or other code, enable it with 'u.add_enabled_units'. For details, see https://docs.astropy.org/en/latest/units/combining_and_defining.html [astropy.units.core]
spec_tbl
WAVELENGTH | SIGNAL | MASK | QUALITY | VAR | NDITH |
---|---|---|---|---|---|
Angstrom | erg / (Angstrom s cm2) | Number | Number | erg2 / (Angstrom2 s2 cm4) | Number |
float32 | float32 | int32 | float32 | float32 | int16 |
11900.0 | 0.0 | 1 | 0.0 | 0.0 | 0 |
11913.4 | 0.0 | 1 | 0.0 | 0.0 | 0 |
11926.8 | 0.0 | 1 | 0.0 | 0.0 | 0 |
11940.2 | 0.0 | 1 | 0.0 | 0.0 | 0 |
11953.6 | 533.2775 | 67 | 0.5919007 | 6.148728 | 1 |
11967.0 | 0.0 | 1 | 0.0 | 0.0 | 0 |
11980.4 | 450.44067 | 65 | 0.99010026 | 2.4839582 | 1 |
11993.8 | 253.39111 | 65 | 0.7950632 | 0.724125 | 1 |
12007.2 | 0.0 | 1 | 0.0 | 0.0 | 0 |
... | ... | ... | ... | ... | ... |
18894.8 | 106.68797 | 64 | 0.875391 | 0.063682236 | 2 |
18908.2 | 117.300804 | 64 | 0.98155046 | 0.08156926 | 2 |
18921.6 | 132.819 | 66 | 0.87731457 | 0.034148376 | 4 |
18935.0 | 139.23103 | 64 | 0.98957026 | 0.057952277 | 4 |
18948.4 | 126.06506 | 64 | 0.74300086 | 0.091131285 | 3 |
18961.8 | 128.77884 | 64 | 0.72368777 | 0.10531768 | 3 |
18975.2 | 131.90378 | 64 | 0.7313887 | 0.14362255 | 3 |
18988.6 | 0.0 | 1 | 0.0 | 0.0 | 0 |
19002.0 | 126.94632 | 66 | 0.49234724 | 0.8100565 | 2 |
plt.plot(spec_tbl['WAVELENGTH'], spec_tbl['SIGNAL'])
plt.xlabel(spec_tbl['WAVELENGTH'].unit.to_string('latex_inline'))
plt.ylabel(spec_tbl['SIGNAL'].unit.to_string('latex_inline'))
plt.title(f'Spectrum of Target: {target_name}\n(Euclid Object ID: {object_id})');
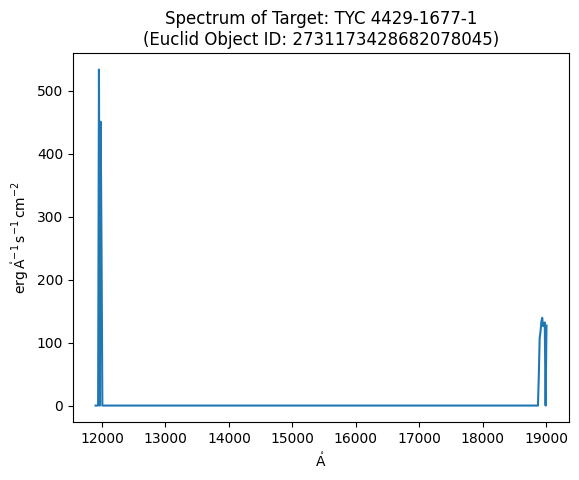
About this Notebook#
Author: Jaladh Singhal (IRSA Developer) in conjunction with Vandana Desai, Brigitta Sipőcz, Tiffany Meshkat and the IPAC Science Platform team
Updated: 2025-03-17
Contact: the IRSA Helpdesk with questions or reporting problems.